.\n",
"\n",
"prefix: a senpy:Entry ;\n",
" nif:isString \"Senpy is the best framework for semantic sentiment analysis, and very easy to use\" ;\n",
" marl:hasOpinion [ a senpy:Sentiment ;\n",
" marl:hasPolarity \"marl:Positive\" ;\n",
" prov:wasGeneratedBy prefix:Analysis_1554364668.5153766 ] .\n",
"\n",
"[] a senpy:Results ;\n",
" prov:used prefix: .\n"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/sentiment140',\n",
" params={\"input\": \"Senpy is the best framework for semantic sentiment analysis, and very easy to use\",\n",
" \"outformat\": \"turtle\"})\n",
"pretty(res.text, language='turtle')"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Selecting fields from the output"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The full output in the previous sections is very useful because it is semantically annotated.\n",
"However, it is also quite verbose if we only want to label a piece of text, or get a polarity value.\n",
"\n",
"For such simple cases, the API has a special `fields` method you can use to get a specific field from the results, and even transform the results. Senpy uses jmespath under the hood, which has its own notation.\n",
"\n",
"To illustrate this, let us get only the text (`nif:isString`) from each entry:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"[\"Senpy is a wonderful service\"]\n"
]
}
],
"source": [
"res = requests.get(f'{endpoint}/sentiment140',\n",
" params={\"input\": \"Senpy is a wonderful service\",\n",
" \"fields\": 'entries[].\"nif:isString\"'})\n",
"print(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Or we could get both the text and the polarity of the text (assuming there is only one opinion per entry) with a slightly more complicated query:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"[\"Senpy is a service. Wonderful service.\", \"marl:Neutral\"]\n"
]
}
],
"source": [
"res = requests.get(f'{endpoint}/sentiment140',\n",
" params={\"input\": \"Senpy is a service. Wonderful service.\",\n",
" \"delimiter\": \"sentence\",\n",
" \"fields\": 'entries[0].[\"nif:isString\", \"marl:hasOpinion\"[0].\"marl:hasPolarity\"]'})\n",
"print(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"jmespath is rather extensive for this tutorial. We will cover only the most simple cases, so you do not need to learn much about the notation.\n",
"\n",
"For more complicated transformations, check out [jmespath](http://jmespath.org).\n",
"In addition to a fairly complete documentation, they have a live environment you can use to test your queries."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Emotion analysis"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"\n",
"Senpy uses the `onyx` vocabulary to represent emotions, which incorporates the notion of `EmotionSet`'s, an emotion that is composed of several emotions.\n",
"In a nutshell, an `Entry` is linked to one or more `EmotionSet`, which in turn is made up of one or more `Emotion`.\n",
"\n",
"Let's illustrate it with an example, using the `emotion-depechemood` plugin."
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tZGVwZWNoZW1vb2Q_aW5wdXQ9U2VucHkraXMrYSt3b25kZXJmdWwrdGhhdCtzZXJ2aWNlIw%3D%3D",\n",
" "@type": "Results",\n",
" "entries": [\n",
" {\n",
" "@id": "prefix:",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [],\n",
" "nif:isString": "Senpy is a wonderful that service",\n",
" "onyx:hasEmotionSet": [\n",
" {\n",
" "@type": "EmotionSet",\n",
" "onyx:hasEmotion": [\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:negative-fear",\n",
" "onyx:hasEmotionIntensity": 0.06258366271018097\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:amusement",\n",
" "onyx:hasEmotionIntensity": 0.15784834034155437\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:anger",\n",
" "onyx:hasEmotionIntensity": 0.08728815135373413\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:annoyance",\n",
" "onyx:hasEmotionIntensity": 0.12184635680460143\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:indifference",\n",
" "onyx:hasEmotionIntensity": 0.1374081151031531\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:joy",\n",
" "onyx:hasEmotionIntensity": 0.12267040802346799\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:awe",\n",
" "onyx:hasEmotionIntensity": 0.21085262130713067\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:sadness",\n",
" "onyx:hasEmotionIntensity": 0.09950234435617733\n",
" }\n",
" ],\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364674.7078097"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tZGVwZWNoZW1vb2Q\\PYZus{}aW5wdXQ9U2VucHkraXMrYSt3b25kZXJmdWwrdGhhdCtzZXJ2aWNlIw\\PYZpc{}3D\\PYZpc{}3D\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Results\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}entries\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is a wonderful that service\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}EmotionSet\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:negative\\PYZhy{}fear\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.06258366271018097}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:amusement\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.15784834034155437}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:anger\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.08728815135373413}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:annoyance\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.12184635680460143}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:indifference\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.1374081151031531}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:joy\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.12267040802346799}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:awe\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.21085262130713067}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:sadness\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.09950234435617733}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364674.7078097\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tZGVwZWNoZW1vb2Q_aW5wdXQ9U2VucHkraXMrYSt3b25kZXJmdWwrdGhhdCtzZXJ2aWNlIw%3D%3D\",\n",
" \"@type\": \"Results\",\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [],\n",
" \"nif:isString\": \"Senpy is a wonderful that service\",\n",
" \"onyx:hasEmotionSet\": [\n",
" {\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:negative-fear\",\n",
" \"onyx:hasEmotionIntensity\": 0.06258366271018097\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:amusement\",\n",
" \"onyx:hasEmotionIntensity\": 0.15784834034155437\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:anger\",\n",
" \"onyx:hasEmotionIntensity\": 0.08728815135373413\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:annoyance\",\n",
" \"onyx:hasEmotionIntensity\": 0.12184635680460143\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:indifference\",\n",
" \"onyx:hasEmotionIntensity\": 0.1374081151031531\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:joy\",\n",
" \"onyx:hasEmotionIntensity\": 0.12267040802346799\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:awe\",\n",
" \"onyx:hasEmotionIntensity\": 0.21085262130713067\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:sadness\",\n",
" \"onyx:hasEmotionIntensity\": 0.09950234435617733\n",
" }\n",
" ],\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364674.7078097\"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/emotion-depechemood',\n",
" params={\"input\": \"Senpy is a wonderful that service\"})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"As you have probably noticed, there are several emotions in this result, each with a different intensity.\n",
"\n",
"We can also tell senpy to only return the emotion with the maximum intensity using the `maxemotion` parameter:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tZGVwZWNoZW1vb2Q_aW5wdXQ9U2VucHkraXMrYSt3b25kZXJmdWwrc2VydmljZSZtYXhlbW90aW9uPVRydWUj",\n",
" "@type": "Results",\n",
" "entries": [\n",
" {\n",
" "@id": "prefix:",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [],\n",
" "nif:isString": "Senpy is a wonderful service",\n",
" "onyx:hasEmotionSet": [\n",
" {\n",
" "@type": "EmotionSet",\n",
" "onyx:hasEmotion": [\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:awe",\n",
" "onyx:hasEmotionIntensity": 0.21085262130713067\n",
" }\n",
" ],\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364674.8374224"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tZGVwZWNoZW1vb2Q\\PYZus{}aW5wdXQ9U2VucHkraXMrYSt3b25kZXJmdWwrc2VydmljZSZtYXhlbW90aW9uPVRydWUj\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Results\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}entries\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is a wonderful service\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}EmotionSet\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:awe\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.21085262130713067}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364674.8374224\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tZGVwZWNoZW1vb2Q_aW5wdXQ9U2VucHkraXMrYSt3b25kZXJmdWwrc2VydmljZSZtYXhlbW90aW9uPVRydWUj\",\n",
" \"@type\": \"Results\",\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [],\n",
" \"nif:isString\": \"Senpy is a wonderful service\",\n",
" \"onyx:hasEmotionSet\": [\n",
" {\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:awe\",\n",
" \"onyx:hasEmotionIntensity\": 0.21085262130713067\n",
" }\n",
" ],\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364674.8374224\"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/emotion-depechemood',\n",
" params={\"input\": \"Senpy is a wonderful service\",\n",
" \"maxemotion\": True})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"We can combine this feature with the `fields` parameter to get only the label and the intensity:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"[["wna:awe", 0.21085262130713067]]\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{[}\\PY{p}{[}\\PY{l+s+s2}{\\PYZdq{}wna:awe\\PYZdq{}}\\PY{p}{,} \\PY{l+m+mf}{0.21085262130713067}\\PY{p}{]}\\PY{p}{]}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"[[\"wna:awe\", 0.21085262130713067]]"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/emotion-depechemood',\n",
" params={\"input\": \"Senpy is a wonderful service\",\n",
" \"fields\": 'entries[].\"onyx:hasEmotionSet\"[].\"onyx:hasEmotion\"[][\"onyx:hasEmotionCategory\",\"onyx:hasEmotionIntensity\"]',\n",
" \"maxemotion\": True})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Emotion conversion"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"If the model used by a plugin is not right for your application, you can ask for a specific emotion model in your request.\n",
"\n",
"Senpy ships with emotion conversion capabilities, and it will try to automatically convert the results.\n",
"\n",
"For example, the `emotion-anew` plugin uses the dimensional `pad` (or VAD, valence-arousal-dominance) model, as we can see here:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tYW5ldz9pbnB1dD1TZW5weStpcythK3dvbmRlcmZ1bCtzZXJ2aWNlK2FuZCtJK2xvdmUraXQj\",\n",
" \"@type\": \"Results\",\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [],\n",
" \"nif:isString\": \"Senpy is a wonderful service and I love it\",\n",
" \"onyx:hasEmotionSet\": [\n",
" {\n",
" \"@id\": \"Emotions0\",\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@id\": \"Emotion0\",\n",
" \"@type\": \"Emotion\",\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#arousal\": 6.44,\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#dominance\": 7.11,\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#valence\": 8.72,\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.1427004\"\n",
" }\n",
" ],\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.1427004\"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}\n"
]
}
],
"source": [
"res = requests.get(f'{endpoint}/emotion-anew',\n",
" params={\"input\": \"Senpy is a wonderful service and I love it\"})\n",
"print(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"If we need a category level, we can ask for the equivalent results in the `big6` model:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tYW5ldz9pbnB1dD1TZW5weStpcythK3dvbmRlcmZ1bCtzZXJ2aWNlK2FuZCtJK2xvdmUraXQmZW1vdGlvbi1tb2RlbD1lbW9tbCUzQWJpZzYj",\n",
" "@type": "Results",\n",
" "entries": [\n",
" {\n",
" "@id": "prefix:",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [],\n",
" "nif:isString": "Senpy is a wonderful service and I love it",\n",
" "onyx:hasEmotionSet": [\n",
" {\n",
" "@id": "Emotions0",\n",
" "@type": "EmotionSet",\n",
" "onyx:hasEmotion": [\n",
" {\n",
" "@id": "Emotion0",\n",
" "@type": "Emotion",\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#arousal": 6.44,\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#dominance": 7.11,\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#valence": 8.72,\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364675.2834926"\n",
" }\n",
" ],\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364675.2834926"\n",
" },\n",
" {\n",
" "@type": "EmotionSet",\n",
" "onyx:hasEmotion": [\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:algorithmConfidence": 7.449999999999999,\n",
" "onyx:hasEmotionCategory": "emoml:big6fear"\n",
" }\n",
" ],\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364675.2902758"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tYW5ldz9pbnB1dD1TZW5weStpcythK3dvbmRlcmZ1bCtzZXJ2aWNlK2FuZCtJK2xvdmUraXQmZW1vdGlvbi1tb2RlbD1lbW9tbCUzQWJpZzYj\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Results\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}entries\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is a wonderful service and I love it\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotions0\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}EmotionSet\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion0\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}arousal\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{6.44}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}dominance\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{7.11}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}valence\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{8.72}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364675.2834926\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364675.2834926\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}EmotionSet\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:algorithmConfidence\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{7.449999999999999}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}emoml:big6fear\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364675.2902758\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tYW5ldz9pbnB1dD1TZW5weStpcythK3dvbmRlcmZ1bCtzZXJ2aWNlK2FuZCtJK2xvdmUraXQmZW1vdGlvbi1tb2RlbD1lbW9tbCUzQWJpZzYj\",\n",
" \"@type\": \"Results\",\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [],\n",
" \"nif:isString\": \"Senpy is a wonderful service and I love it\",\n",
" \"onyx:hasEmotionSet\": [\n",
" {\n",
" \"@id\": \"Emotions0\",\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@id\": \"Emotion0\",\n",
" \"@type\": \"Emotion\",\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#arousal\": 6.44,\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#dominance\": 7.11,\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#valence\": 8.72,\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.2834926\"\n",
" }\n",
" ],\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.2834926\"\n",
" },\n",
" {\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:algorithmConfidence\": 7.449999999999999,\n",
" \"onyx:hasEmotionCategory\": \"emoml:big6fear\"\n",
" }\n",
" ],\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.2902758\"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/emotion-anew',\n",
" params={\"input\": \"Senpy is a wonderful service and I love it\",\n",
" \"emotion-model\": \"emoml:big6\"})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Because we don't usually care about the original emotion, the conversion can be presented in three ways:\n",
"\n",
"* full: the original and converted emotions are included at the same level\n",
"* filtered: the original emotion is replaced by the converted emotion\n",
"* nested: the original emotion is replaced, but the converted emotion points to it\n",
"\n",
"For example, here's how the `nested` structure would look like:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tYW5ldz9pbnB1dD1TZW5weStpcythK3dvbmRlcmZ1bCtzZXJ2aWNlK2FuZCtJK2xvdmUraXQmZW1vdGlvbi1tb2RlbD1lbW9tbCUzQWJpZzYmY29udmVyc2lvbj1uZXN0ZWQj",\n",
" "@type": "Results",\n",
" "entries": [\n",
" {\n",
" "@id": "prefix:",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [],\n",
" "nif:isString": "Senpy is a wonderful service and I love it",\n",
" "onyx:hasEmotionSet": [\n",
" {\n",
" "@type": "EmotionSet",\n",
" "onyx:hasEmotion": [\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:algorithmConfidence": 7.449999999999999,\n",
" "onyx:hasEmotionCategory": "emoml:big6fear"\n",
" }\n",
" ],\n",
" "prov:wasDerivedFrom": {\n",
" "@id": "Emotions0",\n",
" "@type": "EmotionSet",\n",
" "onyx:hasEmotion": [\n",
" {\n",
" "@id": "Emotion0",\n",
" "@type": "Emotion",\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#arousal": 6.44,\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#dominance": 7.11,\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#valence": 8.72,\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364675.4125388"\n",
" }\n",
" ],\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364675.4125388"\n",
" },\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364675.4143574"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tYW5ldz9pbnB1dD1TZW5weStpcythK3dvbmRlcmZ1bCtzZXJ2aWNlK2FuZCtJK2xvdmUraXQmZW1vdGlvbi1tb2RlbD1lbW9tbCUzQWJpZzYmY29udmVyc2lvbj1uZXN0ZWQj\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Results\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}entries\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is a wonderful service and I love it\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}EmotionSet\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:algorithmConfidence\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{7.449999999999999}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}emoml:big6fear\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasDerivedFrom\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotions0\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}EmotionSet\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion0\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}arousal\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{6.44}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}dominance\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{7.11}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}valence\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{8.72}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364675.4125388\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364675.4125388\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364675.4143574\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL2Vtb3Rpb24tYW5ldz9pbnB1dD1TZW5weStpcythK3dvbmRlcmZ1bCtzZXJ2aWNlK2FuZCtJK2xvdmUraXQmZW1vdGlvbi1tb2RlbD1lbW9tbCUzQWJpZzYmY29udmVyc2lvbj1uZXN0ZWQj\",\n",
" \"@type\": \"Results\",\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [],\n",
" \"nif:isString\": \"Senpy is a wonderful service and I love it\",\n",
" \"onyx:hasEmotionSet\": [\n",
" {\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:algorithmConfidence\": 7.449999999999999,\n",
" \"onyx:hasEmotionCategory\": \"emoml:big6fear\"\n",
" }\n",
" ],\n",
" \"prov:wasDerivedFrom\": {\n",
" \"@id\": \"Emotions0\",\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@id\": \"Emotion0\",\n",
" \"@type\": \"Emotion\",\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#arousal\": 6.44,\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#dominance\": 7.11,\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#valence\": 8.72,\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.4125388\"\n",
" }\n",
" ],\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.4125388\"\n",
" },\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.4143574\"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/emotion-anew',\n",
" params={\"input\": \"Senpy is a wonderful service and I love it\",\n",
" \"emotion-model\": \"emoml:big6\",\n",
" \"conversion\": \"nested\"})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Again, for completion, we could get only the label with the `fields` parameter:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"[["Senpy is a wonderful service and I love it", "emoml:big6fear"]]\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{[}\\PY{p}{[}\\PY{l+s+s2}{\\PYZdq{}Senpy is a wonderful service and I love it\\PYZdq{}}\\PY{p}{,} \\PY{l+s+s2}{\\PYZdq{}emoml:big6fear\\PYZdq{}}\\PY{p}{]}\\PY{p}{]}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"[[\"Senpy is a wonderful service and I love it\", \"emoml:big6fear\"]]"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/emotion-anew',\n",
" params={\"input\": \"Senpy is a wonderful service and I love it\",\n",
" \"emotion-model\": \"emoml:big6\",\n",
" \"fields\": 'entries[].[[\"nif:isString\",\"onyx:hasEmotionSet\"[].\"onyx:hasEmotion\"[].\"onyx:hasEmotionCategory\"][]][]',\n",
" \"conversion\": \"filtered\"})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Built-in client"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The built-in senpy client allows you to query any Senpy endpoint. We will illustrate how to use it with the public demo endpoint, and then show you how to spin up your own endpoint using docker."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Building pipelines"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"You can query several senpy services in the same request.\n",
"This feature is called pipelining, and the result of combining several plugins in a request is called a pipeline.\n",
"\n",
"The simplest way to use pipelines is to add every plugin you want to use to the URL, separated by either a slash or a comma.\n",
"\n",
"For instance, to get sentiment (`sentiment140`) and emotion (`depechemood`) annotations at the same time:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpL3NlbnRpbWVudDE0MC9lbW90aW9uLWRlcGVjaGVtb29kP2lucHV0PVNlbnB5K2lzK2Erd29uZGVyZnVsK3NlcnZpY2Uj",\n",
" "@type": "Results",\n",
" "entries": [\n",
" {\n",
" "@id": "prefix:",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [\n",
" {\n",
" "@type": "Sentiment",\n",
" "marl:hasPolarity": "marl:Neutral",\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364675.8928602"\n",
" }\n",
" ],\n",
" "nif:isString": "Senpy is a wonderful service",\n",
" "onyx:hasEmotionSet": [\n",
" {\n",
" "@type": "EmotionSet",\n",
" "onyx:hasEmotion": [\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:negative-fear",\n",
" "onyx:hasEmotionIntensity": 0.06258366271018097\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:amusement",\n",
" "onyx:hasEmotionIntensity": 0.15784834034155437\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:anger",\n",
" "onyx:hasEmotionIntensity": 0.08728815135373413\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:annoyance",\n",
" "onyx:hasEmotionIntensity": 0.12184635680460143\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:indifference",\n",
" "onyx:hasEmotionIntensity": 0.1374081151031531\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:joy",\n",
" "onyx:hasEmotionIntensity": 0.12267040802346799\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:awe",\n",
" "onyx:hasEmotionIntensity": 0.21085262130713067\n",
" },\n",
" {\n",
" "@type": "Emotion",\n",
" "onyx:hasEmotionCategory": "wna:sadness",\n",
" "onyx:hasEmotionIntensity": 0.09950234435617733\n",
" }\n",
" ],\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364675.8937423"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpL3NlbnRpbWVudDE0MC9lbW90aW9uLWRlcGVjaGVtb29kP2lucHV0PVNlbnB5K2lzK2Erd29uZGVyZnVsK3NlcnZpY2Uj\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Results\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}entries\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Sentiment\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasPolarity\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}marl:Neutral\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364675.8928602\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is a wonderful service\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}EmotionSet\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:negative\\PYZhy{}fear\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.06258366271018097}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:amusement\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.15784834034155437}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:anger\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.08728815135373413}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:annoyance\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.12184635680460143}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:indifference\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.1374081151031531}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:joy\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.12267040802346799}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:awe\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.21085262130713067}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionCategory\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}wna:sadness\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionIntensity\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.09950234435617733}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364675.8937423\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL3NlbnRpbWVudDE0MC9lbW90aW9uLWRlcGVjaGVtb29kP2lucHV0PVNlbnB5K2lzK2Erd29uZGVyZnVsK3NlcnZpY2Uj\",\n",
" \"@type\": \"Results\",\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [\n",
" {\n",
" \"@type\": \"Sentiment\",\n",
" \"marl:hasPolarity\": \"marl:Neutral\",\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.8928602\"\n",
" }\n",
" ],\n",
" \"nif:isString\": \"Senpy is a wonderful service\",\n",
" \"onyx:hasEmotionSet\": [\n",
" {\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:negative-fear\",\n",
" \"onyx:hasEmotionIntensity\": 0.06258366271018097\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:amusement\",\n",
" \"onyx:hasEmotionIntensity\": 0.15784834034155437\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:anger\",\n",
" \"onyx:hasEmotionIntensity\": 0.08728815135373413\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:annoyance\",\n",
" \"onyx:hasEmotionIntensity\": 0.12184635680460143\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:indifference\",\n",
" \"onyx:hasEmotionIntensity\": 0.1374081151031531\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:joy\",\n",
" \"onyx:hasEmotionIntensity\": 0.12267040802346799\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:awe\",\n",
" \"onyx:hasEmotionIntensity\": 0.21085262130713067\n",
" },\n",
" {\n",
" \"@type\": \"Emotion\",\n",
" \"onyx:hasEmotionCategory\": \"wna:sadness\",\n",
" \"onyx:hasEmotionIntensity\": 0.09950234435617733\n",
" }\n",
" ],\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364675.8937423\"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/sentiment140/emotion-depechemood',\n",
" params={\"input\": \"Senpy is a wonderful service\"})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"In a senpy pipeline, the call is processed by each plugin in sequence.\n",
"The output of a plugin is used as input for the next one.\n",
"\n",
"Pipelines take the same parameters as the plugins they are made of.\n",
"For example, if we want to split the original sentence before analysing its sentiment, we can use a pipeline made out of the `split` and the `sentiment140` plugins.\n",
"\n",
"`split` takes an extra parameter (`delimiter`) to select the type of splitting (by sentence or by paragraph), and `sentiment140` takes a `language` parameter.\n",
"\n",
"This is how the request looks like:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpL3NwbGl0L3NlbnRpbWVudDE0MD9pbnB1dD1TZW5weStpcythd2Vzb21lLitBbmQrc2VydmljZXMrYXJlK2NvbXBvc2FibGUuJmRlbGltaXRlcj1zZW50ZW5jZSZsYW5ndWFnZT1lbiZvdXRmb3JtYXQ9anNvbi1sZCM%3D",\n",
" "@type": "Results",\n",
" "entries": [\n",
" {\n",
" "@id": "prefix:",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [\n",
" {\n",
" "@type": "Sentiment",\n",
" "marl:hasPolarity": "marl:Positive",\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364676.2060485"\n",
" }\n",
" ],\n",
" "nif:isString": "Senpy is awesome. And services are composable.",\n",
" "onyx:hasEmotionSet": []\n",
" },\n",
" {\n",
" "@id": "prefix:#char=0,17",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [\n",
" {\n",
" "@type": "Sentiment",\n",
" "marl:hasPolarity": "marl:Positive",\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364676.2060485"\n",
" }\n",
" ],\n",
" "nif:isString": "Senpy is awesome.",\n",
" "onyx:hasEmotionSet": []\n",
" },\n",
" {\n",
" "@id": "prefix:#char=18,46",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [\n",
" {\n",
" "@type": "Sentiment",\n",
" "marl:hasPolarity": "marl:Neutral",\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364676.2060485"\n",
" }\n",
" ],\n",
" "nif:isString": "And services are composable.",\n",
" "onyx:hasEmotionSet": []\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpL3NwbGl0L3NlbnRpbWVudDE0MD9pbnB1dD1TZW5weStpcythd2Vzb21lLitBbmQrc2VydmljZXMrYXJlK2NvbXBvc2FibGUuJmRlbGltaXRlcj1zZW50ZW5jZSZsYW5ndWFnZT1lbiZvdXRmb3JtYXQ9anNvbi1sZCM\\PYZpc{}3D\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Results\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}entries\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Sentiment\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasPolarity\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}marl:Positive\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364676.2060485\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is awesome. And services are composable.\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZsh{}char=0,17\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Sentiment\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasPolarity\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}marl:Positive\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364676.2060485\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is awesome.\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZsh{}char=18,46\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Sentiment\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasPolarity\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}marl:Neutral\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364676.2060485\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}And services are composable.\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL3NwbGl0L3NlbnRpbWVudDE0MD9pbnB1dD1TZW5weStpcythd2Vzb21lLitBbmQrc2VydmljZXMrYXJlK2NvbXBvc2FibGUuJmRlbGltaXRlcj1zZW50ZW5jZSZsYW5ndWFnZT1lbiZvdXRmb3JtYXQ9anNvbi1sZCM%3D\",\n",
" \"@type\": \"Results\",\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [\n",
" {\n",
" \"@type\": \"Sentiment\",\n",
" \"marl:hasPolarity\": \"marl:Positive\",\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364676.2060485\"\n",
" }\n",
" ],\n",
" \"nif:isString\": \"Senpy is awesome. And services are composable.\",\n",
" \"onyx:hasEmotionSet\": []\n",
" },\n",
" {\n",
" \"@id\": \"prefix:#char=0,17\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [\n",
" {\n",
" \"@type\": \"Sentiment\",\n",
" \"marl:hasPolarity\": \"marl:Positive\",\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364676.2060485\"\n",
" }\n",
" ],\n",
" \"nif:isString\": \"Senpy is awesome.\",\n",
" \"onyx:hasEmotionSet\": []\n",
" },\n",
" {\n",
" \"@id\": \"prefix:#char=18,46\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [\n",
" {\n",
" \"@type\": \"Sentiment\",\n",
" \"marl:hasPolarity\": \"marl:Neutral\",\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364676.2060485\"\n",
" }\n",
" ],\n",
" \"nif:isString\": \"And services are composable.\",\n",
" \"onyx:hasEmotionSet\": []\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/split/sentiment140',\n",
" params={\"input\": \"Senpy is awesome. And services are composable.\", \n",
" \"delimiter\": \"sentence\",\n",
" \"language\": \"en\",\n",
" \"outformat\": \"json-ld\"})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"As you can see, `split` creates two new entries, which are also annotated by `sentiment140`."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Once again, we could use the `fields` parameter to get a list of strings and labels:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"[["Senpy is awesome. And services are composable.", "marl:Positive"], ["Senpy is awesome.", "marl:Positive"], ["And services are composable.", "marl:Neutral"]]\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{[}\\PY{p}{[}\\PY{l+s+s2}{\\PYZdq{}Senpy is awesome. And services are composable.\\PYZdq{}}\\PY{p}{,} \\PY{l+s+s2}{\\PYZdq{}marl:Positive\\PYZdq{}}\\PY{p}{]}\\PY{p}{,} \\PY{p}{[}\\PY{l+s+s2}{\\PYZdq{}Senpy is awesome.\\PYZdq{}}\\PY{p}{,} \\PY{l+s+s2}{\\PYZdq{}marl:Positive\\PYZdq{}}\\PY{p}{]}\\PY{p}{,} \\PY{p}{[}\\PY{l+s+s2}{\\PYZdq{}And services are composable.\\PYZdq{}}\\PY{p}{,} \\PY{l+s+s2}{\\PYZdq{}marl:Neutral\\PYZdq{}}\\PY{p}{]}\\PY{p}{]}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"[[\"Senpy is awesome. And services are composable.\", \"marl:Positive\"], [\"Senpy is awesome.\", \"marl:Positive\"], [\"And services are composable.\", \"marl:Neutral\"]]"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/split/sentiment140',\n",
" params={\"input\": \"Senpy is awesome. And services are composable.\", \n",
" \"delimiter\": \"sentence\",\n",
" \"fields\": 'entries[].[[\"nif:isString\",\"marl:hasOpinion\"[].\"marl:hasPolarity\"][]][]',\n",
" \"language\": \"en\",\n",
" \"outformat\": \"json-ld\"})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Evaluation"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"Sentiment analysis plugins can also be evaluated on a series of pre-defined datasets, using the `gsitk` tool.\n",
"\n",
"For instance, to evaluate the `sentiment-vader` plugin on the `vader` and `sts` datasets, we would simply call:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpL2V2YWx1YXRlLz9hbGdvPXNlbnRpbWVudC12YWRlciZkYXRhc2V0PXZhZGVyJTJDc3RzJm91dGZvcm1hdD1qc29uLWxkIw%3D%3D",\n",
" "@type": "AggregatedEvaluation",\n",
" "senpy:evaluations": [\n",
" {\n",
" "@type": "Evaluation",\n",
" "evaluates": "endpoint:plugins/sentiment-vader_0.1.1__vader",\n",
" "evaluatesOn": "vader",\n",
" "metrics": [\n",
" {\n",
" "@type": "Accuracy",\n",
" "value": 0.6907142857142857\n",
" },\n",
" {\n",
" "@type": "Precision_macro",\n",
" "value": 0.34535714285714286\n",
" },\n",
" {\n",
" "@type": "Recall_macro",\n",
" "value": 0.5\n",
" },\n",
" {\n",
" "@type": "F1_macro",\n",
" "value": 0.40853400929446554\n",
" },\n",
" {\n",
" "@type": "F1_weighted",\n",
" "value": 0.5643605528396403\n",
" },\n",
" {\n",
" "@type": "F1_micro",\n",
" "value": 0.6907142857142857\n",
" },\n",
" {\n",
" "@type": "F1_macro",\n",
" "value": 0.40853400929446554\n",
" }\n",
" ]\n",
" },\n",
" {\n",
" "@type": "Evaluation",\n",
" "evaluates": "endpoint:plugins/sentiment-vader_0.1.1__sts",\n",
" "evaluatesOn": "sts",\n",
" "metrics": [\n",
" {\n",
" "@type": "Accuracy",\n",
" "value": 0.3107177974434612\n",
" },\n",
" {\n",
" "@type": "Precision_macro",\n",
" "value": 0.1553588987217306\n",
" },\n",
" {\n",
" "@type": "Recall_macro",\n",
" "value": 0.5\n",
" },\n",
" {\n",
" "@type": "F1_macro",\n",
" "value": 0.23705926481620407\n",
" },\n",
" {\n",
" "@type": "F1_weighted",\n",
" "value": 0.14731706525451424\n",
" },\n",
" {\n",
" "@type": "F1_micro",\n",
" "value": 0.3107177974434612\n",
" },\n",
" {\n",
" "@type": "F1_macro",\n",
" "value": 0.23705926481620407\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpL2V2YWx1YXRlLz9hbGdvPXNlbnRpbWVudC12YWRlciZkYXRhc2V0PXZhZGVyJTJDc3RzJm91dGZvcm1hdD1qc29uLWxkIw\\PYZpc{}3D\\PYZpc{}3D\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}AggregatedEvaluation\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}senpy:evaluations\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Evaluation\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}evaluates\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}endpoint:plugins/sentiment\\PYZhy{}vader\\PYZus{}0.1.1\\PYZus{}\\PYZus{}vader\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}evaluatesOn\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}vader\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}metrics\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Accuracy\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.6907142857142857}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Precision\\PYZus{}macro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.34535714285714286}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Recall\\PYZus{}macro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.5}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}F1\\PYZus{}macro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.40853400929446554}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}F1\\PYZus{}weighted\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.5643605528396403}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}F1\\PYZus{}micro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.6907142857142857}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}F1\\PYZus{}macro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.40853400929446554}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Evaluation\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}evaluates\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}endpoint:plugins/sentiment\\PYZhy{}vader\\PYZus{}0.1.1\\PYZus{}\\PYZus{}sts\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}evaluatesOn\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}sts\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}metrics\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Accuracy\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.3107177974434612}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Precision\\PYZus{}macro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.1553588987217306}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Recall\\PYZus{}macro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.5}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}F1\\PYZus{}macro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.23705926481620407}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}F1\\PYZus{}weighted\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.14731706525451424}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}F1\\PYZus{}micro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.3107177974434612}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}F1\\PYZus{}macro\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{0.23705926481620407}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL2V2YWx1YXRlLz9hbGdvPXNlbnRpbWVudC12YWRlciZkYXRhc2V0PXZhZGVyJTJDc3RzJm91dGZvcm1hdD1qc29uLWxkIw%3D%3D\",\n",
" \"@type\": \"AggregatedEvaluation\",\n",
" \"senpy:evaluations\": [\n",
" {\n",
" \"@type\": \"Evaluation\",\n",
" \"evaluates\": \"endpoint:plugins/sentiment-vader_0.1.1__vader\",\n",
" \"evaluatesOn\": \"vader\",\n",
" \"metrics\": [\n",
" {\n",
" \"@type\": \"Accuracy\",\n",
" \"value\": 0.6907142857142857\n",
" },\n",
" {\n",
" \"@type\": \"Precision_macro\",\n",
" \"value\": 0.34535714285714286\n",
" },\n",
" {\n",
" \"@type\": \"Recall_macro\",\n",
" \"value\": 0.5\n",
" },\n",
" {\n",
" \"@type\": \"F1_macro\",\n",
" \"value\": 0.40853400929446554\n",
" },\n",
" {\n",
" \"@type\": \"F1_weighted\",\n",
" \"value\": 0.5643605528396403\n",
" },\n",
" {\n",
" \"@type\": \"F1_micro\",\n",
" \"value\": 0.6907142857142857\n",
" },\n",
" {\n",
" \"@type\": \"F1_macro\",\n",
" \"value\": 0.40853400929446554\n",
" }\n",
" ]\n",
" },\n",
" {\n",
" \"@type\": \"Evaluation\",\n",
" \"evaluates\": \"endpoint:plugins/sentiment-vader_0.1.1__sts\",\n",
" \"evaluatesOn\": \"sts\",\n",
" \"metrics\": [\n",
" {\n",
" \"@type\": \"Accuracy\",\n",
" \"value\": 0.3107177974434612\n",
" },\n",
" {\n",
" \"@type\": \"Precision_macro\",\n",
" \"value\": 0.1553588987217306\n",
" },\n",
" {\n",
" \"@type\": \"Recall_macro\",\n",
" \"value\": 0.5\n",
" },\n",
" {\n",
" \"@type\": \"F1_macro\",\n",
" \"value\": 0.23705926481620407\n",
" },\n",
" {\n",
" \"@type\": \"F1_weighted\",\n",
" \"value\": 0.14731706525451424\n",
" },\n",
" {\n",
" \"@type\": \"F1_micro\",\n",
" \"value\": 0.3107177974434612\n",
" },\n",
" {\n",
" \"@type\": \"F1_macro\",\n",
" \"value\": 0.23705926481620407\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"res = requests.get(f'{endpoint}/evaluate',\n",
" params={\"algo\": \"sentiment-vader\",\n",
" \"dataset\": \"vader,sts\",\n",
" 'outformat': 'json-ld'\n",
" })\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"The same results can be visualized as a table in the Web interface:\n",
"\n",
"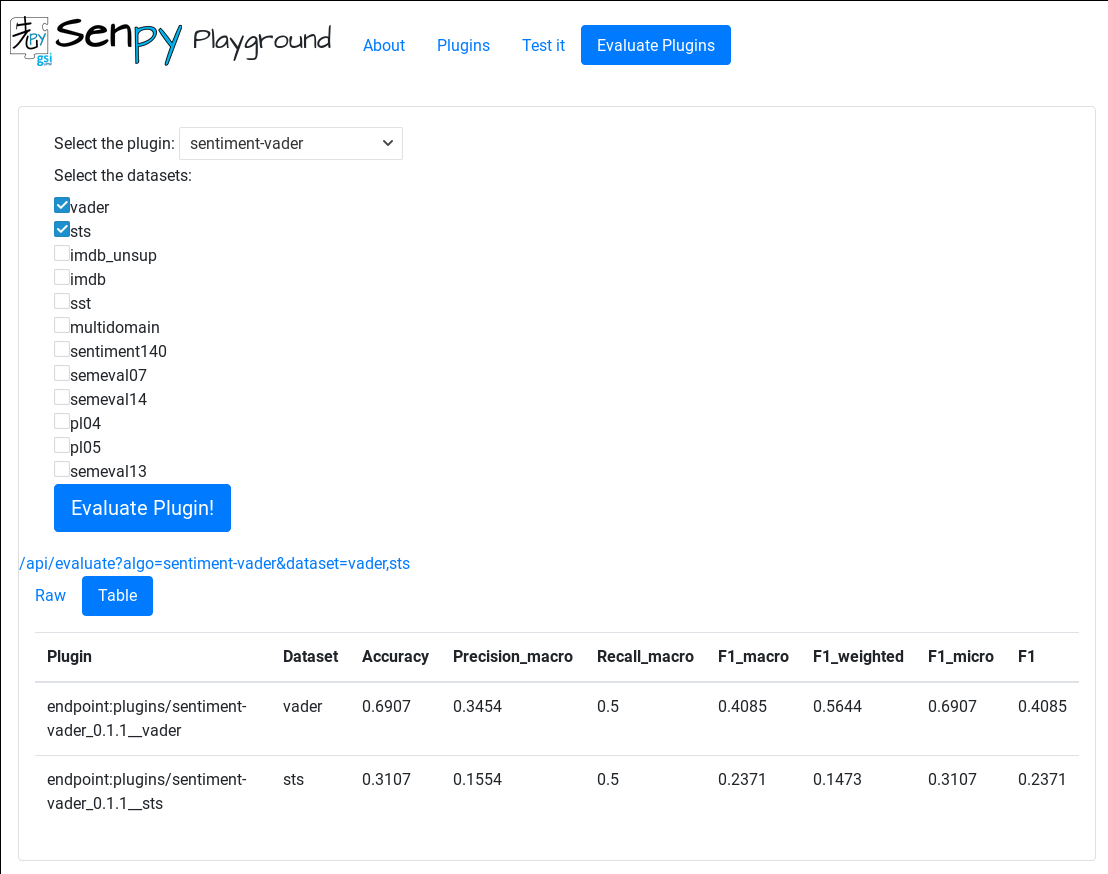"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"**note**: to evaluate a plugin on a dataset, senpy will need to predict the labels of the entries using the plugin.\n",
"This process might take long for plugins that use an external service, such as `sentiment140`."
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"## Advanced topics"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Verbose output"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"By default, senpy does not include information that might be too verbose, such as the parameters that were used in the analysis.\n",
"\n",
"You can instruct senpy to provide a more verbose output with the `verbose` parameter:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpL3NlbnRpbWVudDE0MD9pbnB1dD1TZW5weStpcyt0aGUrYmVzdCtmcmFtZXdvcmsrZm9yK3NlbWFudGljK3NlbnRpbWVudCthbmFseXNpcyUyQythbmQrdmVyeStlYXN5K3RvK3VzZSZ2ZXJib3NlPVRydWUj",\n",
" "@type": "Results",\n",
" "activities": [\n",
" {\n",
" "@id": "prefix:Analysis_1554364688.7944896",\n",
" "@type": "Analysis",\n",
" "marl:maxPolarityValue": 1,\n",
" "marl:minPolarityValue": 0,\n",
" "prov:used": [\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "input",\n",
" "value": "Senpy is the best framework for semantic sentiment analysis, and very easy to use"\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "verbose",\n",
" "value": true\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "in-headers",\n",
" "value": false\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "algorithm",\n",
" "value": "default"\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "expanded-jsonld",\n",
" "value": false\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "with-parameters",\n",
" "value": false\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "outformat",\n",
" "value": "json-ld"\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "help",\n",
" "value": false\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "aliases",\n",
" "value": false\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "conversion",\n",
" "value": "full"\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "intype",\n",
" "value": "direct"\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "informat",\n",
" "value": "text"\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "prefix",\n",
" "value": ""\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "urischeme",\n",
" "value": "RFC5147String"\n",
" },\n",
" {\n",
" "@type": "Parameter",\n",
" "name": "language",\n",
" "value": "auto"\n",
" }\n",
" ],\n",
" "prov:wasAssociatedWith": "endpoint:plugins/sentiment140_0.2"\n",
" }\n",
" ],\n",
" "entries": [\n",
" {\n",
" "@id": "prefix:",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [\n",
" {\n",
" "@type": "Sentiment",\n",
" "marl:hasPolarity": "marl:Positive",\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364688.7944896"\n",
" }\n",
" ],\n",
" "nif:isString": "Senpy is the best framework for semantic sentiment analysis, and very easy to use",\n",
" "onyx:hasEmotionSet": []\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpL3NlbnRpbWVudDE0MD9pbnB1dD1TZW5weStpcyt0aGUrYmVzdCtmcmFtZXdvcmsrZm9yK3NlbWFudGljK3NlbnRpbWVudCthbmFseXNpcyUyQythbmQrdmVyeStlYXN5K3RvK3VzZSZ2ZXJib3NlPVRydWUj\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Results\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}activities\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364688.7944896\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Analysis\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:maxPolarityValue\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mi}{1}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:minPolarityValue\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mi}{0}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:used\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}input\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is the best framework for semantic sentiment analysis, and very easy to use\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}verbose\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}in\\PYZhy{}headers\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}algorithm\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}default\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}expanded\\PYZhy{}jsonld\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}with\\PYZhy{}parameters\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}outformat\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}json\\PYZhy{}ld\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}help\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}conversion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}full\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}intype\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}direct\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}informat\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}text\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}urischeme\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}RFC5147String\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Parameter\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}name\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}language\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}value\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}auto\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasAssociatedWith\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}endpoint:plugins/sentiment140\\PYZus{}0.2\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}entries\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Sentiment\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasPolarity\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}marl:Positive\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364688.7944896\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Senpy is the best framework for semantic sentiment analysis, and very easy to use\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpL3NlbnRpbWVudDE0MD9pbnB1dD1TZW5weStpcyt0aGUrYmVzdCtmcmFtZXdvcmsrZm9yK3NlbWFudGljK3NlbnRpbWVudCthbmFseXNpcyUyQythbmQrdmVyeStlYXN5K3RvK3VzZSZ2ZXJib3NlPVRydWUj\",\n",
" \"@type\": \"Results\",\n",
" \"activities\": [\n",
" {\n",
" \"@id\": \"prefix:Analysis_1554364688.7944896\",\n",
" \"@type\": \"Analysis\",\n",
" \"marl:maxPolarityValue\": 1,\n",
" \"marl:minPolarityValue\": 0,\n",
" \"prov:used\": [\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"input\",\n",
" \"value\": \"Senpy is the best framework for semantic sentiment analysis, and very easy to use\"\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"verbose\",\n",
" \"value\": true\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"in-headers\",\n",
" \"value\": false\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"algorithm\",\n",
" \"value\": \"default\"\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"expanded-jsonld\",\n",
" \"value\": false\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"with-parameters\",\n",
" \"value\": false\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"outformat\",\n",
" \"value\": \"json-ld\"\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"help\",\n",
" \"value\": false\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"aliases\",\n",
" \"value\": false\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"conversion\",\n",
" \"value\": \"full\"\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"intype\",\n",
" \"value\": \"direct\"\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"informat\",\n",
" \"value\": \"text\"\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"prefix\",\n",
" \"value\": \"\"\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"urischeme\",\n",
" \"value\": \"RFC5147String\"\n",
" },\n",
" {\n",
" \"@type\": \"Parameter\",\n",
" \"name\": \"language\",\n",
" \"value\": \"auto\"\n",
" }\n",
" ],\n",
" \"prov:wasAssociatedWith\": \"endpoint:plugins/sentiment140_0.2\"\n",
" }\n",
" ],\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [\n",
" {\n",
" \"@type\": \"Sentiment\",\n",
" \"marl:hasPolarity\": \"marl:Positive\",\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364688.7944896\"\n",
" }\n",
" ],\n",
" \"nif:isString\": \"Senpy is the best framework for semantic sentiment analysis, and very easy to use\",\n",
" \"onyx:hasEmotionSet\": []\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"import requests\n",
"res = requests.get(f'{endpoint}/sentiment140',\n",
" params={\n",
" \"input\": \"Senpy is the best framework for semantic sentiment analysis, and very easy to use\",\n",
" \"verbose\": True}).text\n",
"pretty(res)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Getting help"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@context": "http://senpy.gsi.upm.es/api/contexts/YXBpLz9oZWxwPVRydWUj",\n",
" "@type": "Help",\n",
" "valid_parameters": {\n",
" "algorithm": {\n",
" "aliases": [\n",
" "algorithms",\n",
" "a",\n",
" "algo"\n",
" ],\n",
" "default": "default",\n",
" "description": "Algorithms that will be used to process the request.It may be a list of comma-separated names.",\n",
" "processor": "string_to_tuple",\n",
" "required": true\n",
" },\n",
" "aliases": {\n",
" "@id": "aliases",\n",
" "aliases": [],\n",
" "default": false,\n",
" "description": "Replace JSON properties with their aliases",\n",
" "options": [\n",
" true,\n",
" false\n",
" ],\n",
" "required": true\n",
" },\n",
" "conversion": {\n",
" "@id": "conversion",\n",
" "default": "full",\n",
" "description": "How to show the elements that have (not) been converted.\\n\\n* full: converted and original elements will appear side-by-side\\n* filtered: only converted elements will be shown\\n* nested: converted elements will be shown, and they will include a link to the original element\\n(using `prov:wasGeneratedBy`).\\n",\n",
" "options": [\n",
" "filtered",\n",
" "nested",\n",
" "full"\n",
" ],\n",
" "required": true\n",
" },\n",
" "emotion-model": {\n",
" "@id": "emotionModel",\n",
" "aliases": [\n",
" "emoModel",\n",
" "emotionModel"\n",
" ],\n",
" "description": "Emotion model to use in the response.\\nSenpy will try to convert the output to this model automatically.\\n\\nExamples: `wna:liking` and `emoml:big6`.\\n ",\n",
" "required": false\n",
" },\n",
" "expanded-jsonld": {\n",
" "@id": "expanded-jsonld",\n",
" "aliases": [\n",
" "expanded",\n",
" "expanded_jsonld"\n",
" ],\n",
" "default": false,\n",
" "description": "use JSON-LD expansion to get full URIs",\n",
" "options": [\n",
" true,\n",
" false\n",
" ],\n",
" "required": true\n",
" },\n",
" "fields": {\n",
" "@id": "fields",\n",
" "description": "A jmespath selector, that can be used to extract a new dictionary, array or value\\nfrom the results.\\njmespath is a powerful query language for json and/or dictionaries.\\nIt allows you to change the structure (and data) of your objects through queries.\\n\\ne.g., the following expression gets a list of `[emotion label, intensity]` for each entry:\\n`entries[].\\"onyx:hasEmotionSet\\"[].\\"onyx:hasEmotion\\"[][\\"onyx:hasEmotionCategory\\",\\"onyx:hasEmotionIntensity\\"]`\\n\\nFor more information, see: https://jmespath.org\\n\\n",\n",
" "required": false\n",
" },\n",
" "help": {\n",
" "@id": "help",\n",
" "aliases": [\n",
" "h"\n",
" ],\n",
" "default": false,\n",
" "description": "Show additional help to know more about the possible parameters",\n",
" "options": [\n",
" true,\n",
" false\n",
" ],\n",
" "required": true\n",
" },\n",
" "in-headers": {\n",
" "aliases": [\n",
" "headers",\n",
" "inheaders",\n",
" "inHeaders",\n",
" "in-headers",\n",
" "in_headers"\n",
" ],\n",
" "default": false,\n",
" "description": "Only include the JSON-LD context in the headers",\n",
" "options": [\n",
" true,\n",
" false\n",
" ],\n",
" "required": true\n",
" },\n",
" "informat": {\n",
" "@id": "informat",\n",
" "aliases": [\n",
" "f"\n",
" ],\n",
" "default": "text",\n",
" "description": "input format",\n",
" "options": [\n",
" "text",\n",
" "json-ld"\n",
" ],\n",
" "required": false\n",
" },\n",
" "input": {\n",
" "@id": "input",\n",
" "aliases": [\n",
" "i"\n",
" ],\n",
" "help": "Input text",\n",
" "required": true\n",
" },\n",
" "intype": {\n",
" "@id": "intype",\n",
" "aliases": [\n",
" "t"\n",
" ],\n",
" "default": "direct",\n",
" "description": "input type",\n",
" "options": [\n",
" "direct",\n",
" "url",\n",
" "file"\n",
" ],\n",
" "required": false\n",
" },\n",
" "language": {\n",
" "aliases": [\n",
" "language",\n",
" "l"\n",
" ],\n",
" "default": "en",\n",
" "description": "language of the input",\n",
" "options": [\n",
" "es",\n",
" "en"\n",
" ],\n",
" "required": true\n",
" },\n",
" "outformat": {\n",
" "@id": "outformat",\n",
" "aliases": [\n",
" "o"\n",
" ],\n",
" "default": "json-ld",\n",
" "description": "The data can be semantically formatted (JSON-LD, turtle or n-triples),\\ngiven as a list of comma-separated fields (see the fields option) or constructed from a Jinja2\\ntemplate (see the template option).",\n",
" "options": [\n",
" "json-ld",\n",
" "turtle",\n",
" "ntriples"\n",
" ],\n",
" "required": true\n",
" },\n",
" "prefix": {\n",
" "@id": "prefix",\n",
" "aliases": [\n",
" "p"\n",
" ],\n",
" "default": "",\n",
" "description": "prefix to use for new entities",\n",
" "required": true\n",
" },\n",
" "template": {\n",
" "@id": "template",\n",
" "description": "Jinja2 template for the result. The input data for the template will\\nbe the results as a dictionary.\\nFor example:\\n\\nConsider the results before templating:\\n\\n```\\n[{\\n \\"@type\\": \\"entry\\",\\n \\"onyx:hasEmotionSet\\": [],\\n \\"nif:isString\\": \\"testing the template\\",\\n \\"marl:hasOpinion\\": [\\n {\\n \\"@type\\": \\"sentiment\\",\\n \\"marl:hasPolarity\\": \\"marl:Positive\\"\\n }\\n ]\\n}]\\n```\\n\\n\\nAnd the template:\\n\\n```\\n{% for entry in entries %}\\n{{ entry[\\"nif:isString\\"] | upper }},{{entry.sentiments[0][\\"marl:hasPolarity\\"].split(\\":\\")[1]}}\\n{% endfor %}\\n```\\n\\nThe final result would be:\\n\\n```\\nTESTING THE TEMPLATE,Positive\\n```\\n",\n",
" "required": false\n",
" },\n",
" "urischeme": {\n",
" "@id": "urischeme",\n",
" "aliases": [\n",
" "u"\n",
" ],\n",
" "default": "RFC5147String",\n",
" "description": "scheme for NIF URIs",\n",
" "options": [\n",
" "RFC5147String"\n",
" ],\n",
" "required": false\n",
" },\n",
" "verbose": {\n",
" "@id": "verbose",\n",
" "aliases": [\n",
" "v"\n",
" ],\n",
" "default": false,\n",
" "description": "Show all properties in the result",\n",
" "options": [\n",
" true,\n",
" false\n",
" ],\n",
" "required": true\n",
" },\n",
" "with-parameters": {\n",
" "aliases": [\n",
" "withparameters",\n",
" "with_parameters"\n",
" ],\n",
" "default": false,\n",
" "description": "include initial parameters in the response",\n",
" "options": [\n",
" true,\n",
" false\n",
" ],\n",
" "required": true\n",
" }\n",
" }\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@context\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}http://senpy.gsi.upm.es/api/contexts/YXBpLz9oZWxwPVRydWUj\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Help\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}valid\\PYZus{}parameters\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nt}{\\PYZdq{}algorithm\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}algorithms\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}a\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}algo\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Algorithms that will be used to process the request.It may be a list of comma\\PYZhy{}separated names.\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}processor\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}string\\PYZus{}to\\PYZus{}tuple\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Replace JSON properties with their aliases\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{k+kc}{true}\\PY{p}{,}\n",
" \\PY{k+kc}{false}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}conversion\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}conversion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}full\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}How to show the elements that have (not) been converted.\\PYZbs{}n\\PYZbs{}n* full: converted and original elements will appear side\\PYZhy{}by\\PYZhy{}side\\PYZbs{}n* filtered: only converted elements will be shown\\PYZbs{}n* nested: converted elements will be shown, and they will include a link to the original element\\PYZbs{}n(using `prov:wasGeneratedBy`).\\PYZbs{}n\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}filtered\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}nested\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}full\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}emotion\\PYZhy{}model\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}emotionModel\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}emoModel\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}emotionModel\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion model to use in the response.\\PYZbs{}nSenpy will try to convert the output to this model automatically.\\PYZbs{}n\\PYZbs{}nExamples: `wna:liking` and `emoml:big6`.\\PYZbs{}n \\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}expanded\\PYZhy{}jsonld\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}expanded\\PYZhy{}jsonld\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}expanded\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}expanded\\PYZus{}jsonld\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}use JSON\\PYZhy{}LD expansion to get full URIs\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{k+kc}{true}\\PY{p}{,}\n",
" \\PY{k+kc}{false}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}fields\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}fields\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}A jmespath selector, that can be used to extract a new dictionary, array or value\\PYZbs{}nfrom the results.\\PYZbs{}njmespath is a powerful query language for json and/or dictionaries.\\PYZbs{}nIt allows you to change the structure (and data) of your objects through queries.\\PYZbs{}n\\PYZbs{}ne.g., the following expression gets a list of `[emotion label, intensity]` for each entry:\\PYZbs{}n`entries[].\\PYZbs{}\\PYZdq{}onyx:hasEmotionSet\\PYZbs{}\\PYZdq{}[].\\PYZbs{}\\PYZdq{}onyx:hasEmotion\\PYZbs{}\\PYZdq{}[][\\PYZbs{}\\PYZdq{}onyx:hasEmotionCategory\\PYZbs{}\\PYZdq{},\\PYZbs{}\\PYZdq{}onyx:hasEmotionIntensity\\PYZbs{}\\PYZdq{}]`\\PYZbs{}n\\PYZbs{}nFor more information, see: https://jmespath.org\\PYZbs{}n\\PYZbs{}n\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}help\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}help\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}h\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Show additional help to know more about the possible parameters\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{k+kc}{true}\\PY{p}{,}\n",
" \\PY{k+kc}{false}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}in\\PYZhy{}headers\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}headers\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}inheaders\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}inHeaders\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}in\\PYZhy{}headers\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}in\\PYZus{}headers\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Only include the JSON\\PYZhy{}LD context in the headers\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{k+kc}{true}\\PY{p}{,}\n",
" \\PY{k+kc}{false}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}informat\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}informat\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}f\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}text\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}input format\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}text\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}json\\PYZhy{}ld\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}input\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}input\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}i\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}help\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Input text\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}intype\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}intype\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}t\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}direct\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}input type\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}direct\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}url\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}file\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}language\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}language\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}l\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}en\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}language of the input\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}es\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}en\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}outformat\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}outformat\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}o\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}json\\PYZhy{}ld\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}The data can be semantically formatted (JSON\\PYZhy{}LD, turtle or n\\PYZhy{}triples),\\PYZbs{}ngiven as a list of comma\\PYZhy{}separated fields (see the fields option) or constructed from a Jinja2\\PYZbs{}ntemplate (see the template option).\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}json\\PYZhy{}ld\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}turtle\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}ntriples\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prefix\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}p\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix to use for new entities\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}template\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}template\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Jinja2 template for the result. The input data for the template will\\PYZbs{}nbe the results as a dictionary.\\PYZbs{}nFor example:\\PYZbs{}n\\PYZbs{}nConsider the results before templating:\\PYZbs{}n\\PYZbs{}n```\\PYZbs{}n[\\PYZob{}\\PYZbs{}n \\PYZbs{}\\PYZdq{}@type\\PYZbs{}\\PYZdq{}: \\PYZbs{}\\PYZdq{}entry\\PYZbs{}\\PYZdq{},\\PYZbs{}n \\PYZbs{}\\PYZdq{}onyx:hasEmotionSet\\PYZbs{}\\PYZdq{}: [],\\PYZbs{}n \\PYZbs{}\\PYZdq{}nif:isString\\PYZbs{}\\PYZdq{}: \\PYZbs{}\\PYZdq{}testing the template\\PYZbs{}\\PYZdq{},\\PYZbs{}n \\PYZbs{}\\PYZdq{}marl:hasOpinion\\PYZbs{}\\PYZdq{}: [\\PYZbs{}n \\PYZob{}\\PYZbs{}n \\PYZbs{}\\PYZdq{}@type\\PYZbs{}\\PYZdq{}: \\PYZbs{}\\PYZdq{}sentiment\\PYZbs{}\\PYZdq{},\\PYZbs{}n \\PYZbs{}\\PYZdq{}marl:hasPolarity\\PYZbs{}\\PYZdq{}: \\PYZbs{}\\PYZdq{}marl:Positive\\PYZbs{}\\PYZdq{}\\PYZbs{}n \\PYZcb{}\\PYZbs{}n ]\\PYZbs{}n\\PYZcb{}]\\PYZbs{}n```\\PYZbs{}n\\PYZbs{}n\\PYZbs{}nAnd the template:\\PYZbs{}n\\PYZbs{}n```\\PYZbs{}n\\PYZob{}\\PYZpc{} for entry in entries \\PYZpc{}\\PYZcb{}\\PYZbs{}n\\PYZob{}\\PYZob{} entry[\\PYZbs{}\\PYZdq{}nif:isString\\PYZbs{}\\PYZdq{}] | upper \\PYZcb{}\\PYZcb{},\\PYZob{}\\PYZob{}entry.sentiments[0][\\PYZbs{}\\PYZdq{}marl:hasPolarity\\PYZbs{}\\PYZdq{}].split(\\PYZbs{}\\PYZdq{}:\\PYZbs{}\\PYZdq{})[1]\\PYZcb{}\\PYZcb{}\\PYZbs{}n\\PYZob{}\\PYZpc{} endfor \\PYZpc{}\\PYZcb{}\\PYZbs{}n```\\PYZbs{}n\\PYZbs{}nThe final result would be:\\PYZbs{}n\\PYZbs{}n```\\PYZbs{}nTESTING THE TEMPLATE,Positive\\PYZbs{}n```\\PYZbs{}n\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}urischeme\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}urischeme\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}u\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}RFC5147String\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}scheme for NIF URIs\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}RFC5147String\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}verbose\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}verbose\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}v\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Show all properties in the result\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{k+kc}{true}\\PY{p}{,}\n",
" \\PY{k+kc}{false}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}with\\PYZhy{}parameters\\PYZdq{}}\\PY{p}{:} \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nt}{\\PYZdq{}aliases\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{l+s+s2}{\\PYZdq{}withparameters\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{l+s+s2}{\\PYZdq{}with\\PYZus{}parameters\\PYZdq{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}default\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{false}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}description\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}include initial parameters in the response\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}options\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{k+kc}{true}\\PY{p}{,}\n",
" \\PY{k+kc}{false}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}required\\PYZdq{}}\\PY{p}{:} \\PY{k+kc}{true}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@context\": \"http://senpy.gsi.upm.es/api/contexts/YXBpLz9oZWxwPVRydWUj\",\n",
" \"@type\": \"Help\",\n",
" \"valid_parameters\": {\n",
" \"algorithm\": {\n",
" \"aliases\": [\n",
" \"algorithms\",\n",
" \"a\",\n",
" \"algo\"\n",
" ],\n",
" \"default\": \"default\",\n",
" \"description\": \"Algorithms that will be used to process the request.It may be a list of comma-separated names.\",\n",
" \"processor\": \"string_to_tuple\",\n",
" \"required\": true\n",
" },\n",
" \"aliases\": {\n",
" \"@id\": \"aliases\",\n",
" \"aliases\": [],\n",
" \"default\": false,\n",
" \"description\": \"Replace JSON properties with their aliases\",\n",
" \"options\": [\n",
" true,\n",
" false\n",
" ],\n",
" \"required\": true\n",
" },\n",
" \"conversion\": {\n",
" \"@id\": \"conversion\",\n",
" \"default\": \"full\",\n",
" \"description\": \"How to show the elements that have (not) been converted.\\n\\n* full: converted and original elements will appear side-by-side\\n* filtered: only converted elements will be shown\\n* nested: converted elements will be shown, and they will include a link to the original element\\n(using `prov:wasGeneratedBy`).\\n\",\n",
" \"options\": [\n",
" \"filtered\",\n",
" \"nested\",\n",
" \"full\"\n",
" ],\n",
" \"required\": true\n",
" },\n",
" \"emotion-model\": {\n",
" \"@id\": \"emotionModel\",\n",
" \"aliases\": [\n",
" \"emoModel\",\n",
" \"emotionModel\"\n",
" ],\n",
" \"description\": \"Emotion model to use in the response.\\nSenpy will try to convert the output to this model automatically.\\n\\nExamples: `wna:liking` and `emoml:big6`.\\n \",\n",
" \"required\": false\n",
" },\n",
" \"expanded-jsonld\": {\n",
" \"@id\": \"expanded-jsonld\",\n",
" \"aliases\": [\n",
" \"expanded\",\n",
" \"expanded_jsonld\"\n",
" ],\n",
" \"default\": false,\n",
" \"description\": \"use JSON-LD expansion to get full URIs\",\n",
" \"options\": [\n",
" true,\n",
" false\n",
" ],\n",
" \"required\": true\n",
" },\n",
" \"fields\": {\n",
" \"@id\": \"fields\",\n",
" \"description\": \"A jmespath selector, that can be used to extract a new dictionary, array or value\\nfrom the results.\\njmespath is a powerful query language for json and/or dictionaries.\\nIt allows you to change the structure (and data) of your objects through queries.\\n\\ne.g., the following expression gets a list of `[emotion label, intensity]` for each entry:\\n`entries[].\\\"onyx:hasEmotionSet\\\"[].\\\"onyx:hasEmotion\\\"[][\\\"onyx:hasEmotionCategory\\\",\\\"onyx:hasEmotionIntensity\\\"]`\\n\\nFor more information, see: https://jmespath.org\\n\\n\",\n",
" \"required\": false\n",
" },\n",
" \"help\": {\n",
" \"@id\": \"help\",\n",
" \"aliases\": [\n",
" \"h\"\n",
" ],\n",
" \"default\": false,\n",
" \"description\": \"Show additional help to know more about the possible parameters\",\n",
" \"options\": [\n",
" true,\n",
" false\n",
" ],\n",
" \"required\": true\n",
" },\n",
" \"in-headers\": {\n",
" \"aliases\": [\n",
" \"headers\",\n",
" \"inheaders\",\n",
" \"inHeaders\",\n",
" \"in-headers\",\n",
" \"in_headers\"\n",
" ],\n",
" \"default\": false,\n",
" \"description\": \"Only include the JSON-LD context in the headers\",\n",
" \"options\": [\n",
" true,\n",
" false\n",
" ],\n",
" \"required\": true\n",
" },\n",
" \"informat\": {\n",
" \"@id\": \"informat\",\n",
" \"aliases\": [\n",
" \"f\"\n",
" ],\n",
" \"default\": \"text\",\n",
" \"description\": \"input format\",\n",
" \"options\": [\n",
" \"text\",\n",
" \"json-ld\"\n",
" ],\n",
" \"required\": false\n",
" },\n",
" \"input\": {\n",
" \"@id\": \"input\",\n",
" \"aliases\": [\n",
" \"i\"\n",
" ],\n",
" \"help\": \"Input text\",\n",
" \"required\": true\n",
" },\n",
" \"intype\": {\n",
" \"@id\": \"intype\",\n",
" \"aliases\": [\n",
" \"t\"\n",
" ],\n",
" \"default\": \"direct\",\n",
" \"description\": \"input type\",\n",
" \"options\": [\n",
" \"direct\",\n",
" \"url\",\n",
" \"file\"\n",
" ],\n",
" \"required\": false\n",
" },\n",
" \"language\": {\n",
" \"aliases\": [\n",
" \"language\",\n",
" \"l\"\n",
" ],\n",
" \"default\": \"en\",\n",
" \"description\": \"language of the input\",\n",
" \"options\": [\n",
" \"es\",\n",
" \"en\"\n",
" ],\n",
" \"required\": true\n",
" },\n",
" \"outformat\": {\n",
" \"@id\": \"outformat\",\n",
" \"aliases\": [\n",
" \"o\"\n",
" ],\n",
" \"default\": \"json-ld\",\n",
" \"description\": \"The data can be semantically formatted (JSON-LD, turtle or n-triples),\\ngiven as a list of comma-separated fields (see the fields option) or constructed from a Jinja2\\ntemplate (see the template option).\",\n",
" \"options\": [\n",
" \"json-ld\",\n",
" \"turtle\",\n",
" \"ntriples\"\n",
" ],\n",
" \"required\": true\n",
" },\n",
" \"prefix\": {\n",
" \"@id\": \"prefix\",\n",
" \"aliases\": [\n",
" \"p\"\n",
" ],\n",
" \"default\": \"\",\n",
" \"description\": \"prefix to use for new entities\",\n",
" \"required\": true\n",
" },\n",
" \"template\": {\n",
" \"@id\": \"template\",\n",
" \"description\": \"Jinja2 template for the result. The input data for the template will\\nbe the results as a dictionary.\\nFor example:\\n\\nConsider the results before templating:\\n\\n```\\n[{\\n \\\"@type\\\": \\\"entry\\\",\\n \\\"onyx:hasEmotionSet\\\": [],\\n \\\"nif:isString\\\": \\\"testing the template\\\",\\n \\\"marl:hasOpinion\\\": [\\n {\\n \\\"@type\\\": \\\"sentiment\\\",\\n \\\"marl:hasPolarity\\\": \\\"marl:Positive\\\"\\n }\\n ]\\n}]\\n```\\n\\n\\nAnd the template:\\n\\n```\\n{% for entry in entries %}\\n{{ entry[\\\"nif:isString\\\"] | upper }},{{entry.sentiments[0][\\\"marl:hasPolarity\\\"].split(\\\":\\\")[1]}}\\n{% endfor %}\\n```\\n\\nThe final result would be:\\n\\n```\\nTESTING THE TEMPLATE,Positive\\n```\\n\",\n",
" \"required\": false\n",
" },\n",
" \"urischeme\": {\n",
" \"@id\": \"urischeme\",\n",
" \"aliases\": [\n",
" \"u\"\n",
" ],\n",
" \"default\": \"RFC5147String\",\n",
" \"description\": \"scheme for NIF URIs\",\n",
" \"options\": [\n",
" \"RFC5147String\"\n",
" ],\n",
" \"required\": false\n",
" },\n",
" \"verbose\": {\n",
" \"@id\": \"verbose\",\n",
" \"aliases\": [\n",
" \"v\"\n",
" ],\n",
" \"default\": false,\n",
" \"description\": \"Show all properties in the result\",\n",
" \"options\": [\n",
" true,\n",
" false\n",
" ],\n",
" \"required\": true\n",
" },\n",
" \"with-parameters\": {\n",
" \"aliases\": [\n",
" \"withparameters\",\n",
" \"with_parameters\"\n",
" ],\n",
" \"default\": false,\n",
" \"description\": \"include initial parameters in the response\",\n",
" \"options\": [\n",
" true,\n",
" false\n",
" ],\n",
" \"required\": true\n",
" }\n",
" }\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"import requests\n",
"res = requests.get(f'{endpoint}/',\n",
" params={\n",
" \"help\": True}).text\n",
"pretty(res)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"### Ignoring the context"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"data": {
"text/html": [
"{\n",
" "@type": "Results",\n",
" "entries": [\n",
" {\n",
" "@id": "prefix:",\n",
" "@type": "Entry",\n",
" "marl:hasOpinion": [],\n",
" "nif:isString": "This will tell senpy to only include the context in the headers",\n",
" "onyx:hasEmotionSet": [\n",
" {\n",
" "@id": "Emotions0",\n",
" "@type": "EmotionSet",\n",
" "onyx:hasEmotion": [\n",
" {\n",
" "@id": "Emotion0",\n",
" "@type": "Emotion",\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#arousal": 4.22,\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#dominance": 5.17,\n",
" "http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#valence": 5.2,\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364689.0180304"\n",
" }\n",
" ],\n",
" "prov:wasGeneratedBy": "prefix:Analysis_1554364689.0180304"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}\n",
"
\n"
],
"text/latex": [
"\\begin{Verbatim}[commandchars=\\\\\\{\\}]\n",
"\\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Results\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}entries\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Entry\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}marl:hasOpinion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}nif:isString\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}This will tell senpy to only include the context in the headers\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotionSet\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotions0\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}EmotionSet\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}onyx:hasEmotion\\PYZdq{}}\\PY{p}{:} \\PY{p}{[}\n",
" \\PY{p}{\\PYZob{}}\n",
" \\PY{n+nd}{\\PYZdq{}@id\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion0\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nd}{\\PYZdq{}@type\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}Emotion\\PYZdq{}}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}arousal\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{4.22}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}dominance\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{5.17}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns\\PYZsh{}valence\\PYZdq{}}\\PY{p}{:} \\PY{l+m+mf}{5.2}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364689.0180304\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\\PY{p}{,}\n",
" \\PY{n+nt}{\\PYZdq{}prov:wasGeneratedBy\\PYZdq{}}\\PY{p}{:} \\PY{l+s+s2}{\\PYZdq{}prefix:Analysis\\PYZus{}1554364689.0180304\\PYZdq{}}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
" \\PY{p}{\\PYZcb{}}\n",
" \\PY{p}{]}\n",
"\\PY{p}{\\PYZcb{}}\n",
"\\end{Verbatim}\n"
],
"text/plain": [
"{\n",
" \"@type\": \"Results\",\n",
" \"entries\": [\n",
" {\n",
" \"@id\": \"prefix:\",\n",
" \"@type\": \"Entry\",\n",
" \"marl:hasOpinion\": [],\n",
" \"nif:isString\": \"This will tell senpy to only include the context in the headers\",\n",
" \"onyx:hasEmotionSet\": [\n",
" {\n",
" \"@id\": \"Emotions0\",\n",
" \"@type\": \"EmotionSet\",\n",
" \"onyx:hasEmotion\": [\n",
" {\n",
" \"@id\": \"Emotion0\",\n",
" \"@type\": \"Emotion\",\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#arousal\": 4.22,\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#dominance\": 5.17,\n",
" \"http://www.gsi.dit.upm.es/ontologies/onyx/vocabularies/anew/ns#valence\": 5.2,\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364689.0180304\"\n",
" }\n",
" ],\n",
" \"prov:wasGeneratedBy\": \"prefix:Analysis_1554364689.0180304\"\n",
" }\n",
" ]\n",
" }\n",
" ]\n",
"}"
]
},
"execution_count": null,
"metadata": {},
"output_type": "execute_result"
}
],
"source": [
"import requests\n",
"res = requests.get(f'{endpoint}/',\n",
" params={\n",
" \"input\": \"This will tell senpy to only include the context in the headers\",\n",
" \"inheaders\": True})\n",
"pretty(res.text)"
]
},
{
"cell_type": "markdown",
"metadata": {},
"source": [
"To retrieve the context URI, use the `LINK` header:"
]
},
{
"cell_type": "code",
"execution_count": null,
"metadata": {},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
";rel=\"http://www.w3.org/ns/json-ld#context\"; type=\"application/ld+json\"\n"
]
}
],
"source": [
"print(res.headers['Link'])"
]
}
],
"metadata": {
"anaconda-cloud": {},
"kernelspec": {
"display_name": "Python 3",
"language": "python",
"name": "python3"
},
"language_info": {
"codemirror_mode": {
"name": "ipython",
"version": 3
},
"file_extension": ".py",
"mimetype": "text/x-python",
"name": "python",
"nbconvert_exporter": "python",
"pygments_lexer": "ipython3",
"version": "3.7.3"
},
"toc": {
"colors": {
"hover_highlight": "#DAA520",
"running_highlight": "#FF0000",
"selected_highlight": "#FFD700"
},
"moveMenuLeft": true,
"nav_menu": {
"height": "68px",
"width": "252px"
},
"navigate_menu": true,
"number_sections": true,
"sideBar": true,
"threshold": 4,
"toc_cell": false,
"toc_section_display": "block",
"toc_window_display": false
}
},
"nbformat": 4,
"nbformat_minor": 1
}